Google OAuth2 Authentication using Owin Security in your ASP.NET C# project using just few lines of code
Visual studio scaffolding allows you to generate code to perform Google G-Suite authentication while creating a new ASP.NET project. However, this scaffolding adds a lot of code to your project that you may not require.
I will walk you through the steps required to perform the same without going through the scaffolding process.
STEP 1 Create an empty ASP.NET project in Visual Studio
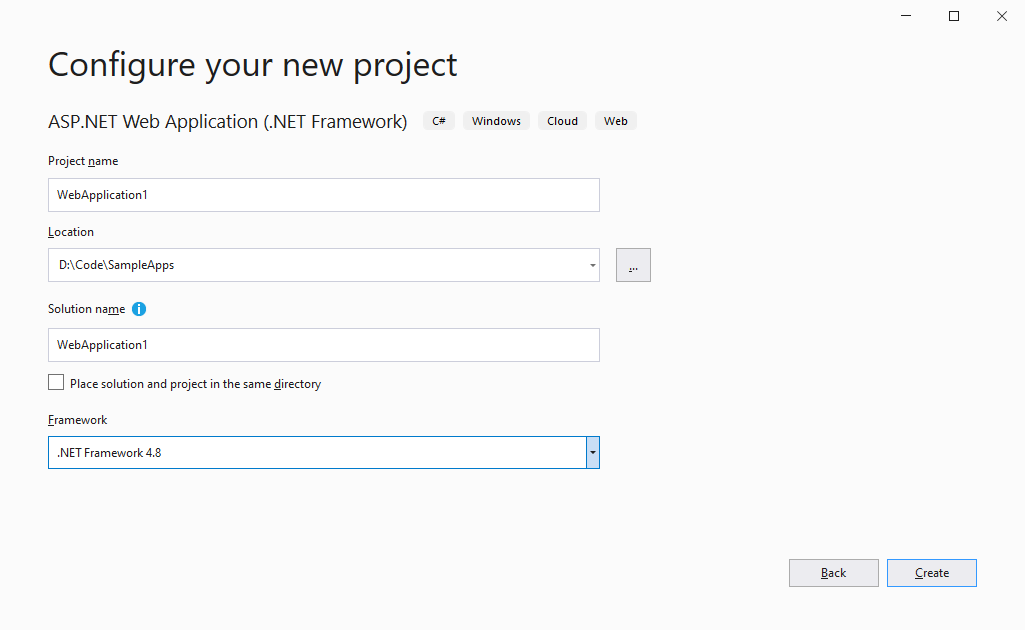
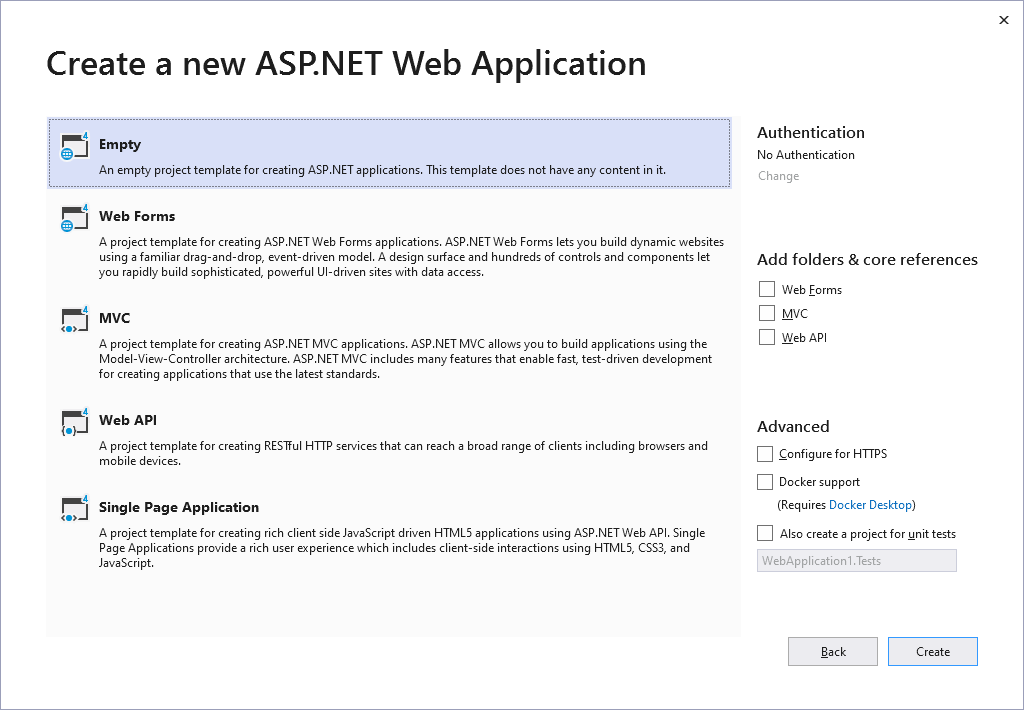
STEP 2 Install the dependencies using nuget Package Manager.
PM> Install-Package Microsoft.AspNet.Identity.Owin PM> Install-Package Microsoft.Owin.Host.SystemWeb PM> Install-Package Microsoft.Owin.Security.Google
STEP 3 Create a service account to be used in G-Suite Authentication
Navigate to https://console.developers.google.com/ and login with your Gmail or G-Suite credentials. Select NEW PROJECT. Give a valid Project Name and click on Create.
After that, navigate to the OAuth consent screen of this Project. Select appropriate User Type and click create. On the next screen, mandatory fields like App name and Email address and click on SAVE AND CONTINUE.
Similarly, navigate to Credentials of this Project. Click on CREATE CREDENTIALS – OAuth client ID. Choose Web Application for the application type. Provide a name for the application.
Authorized JavaScript origin is the URI where your website will be hosted. For a development environment, this URI will be like http://localhost:55293
. Check you Visual Studio project properties for the exact port, as it usually differs for every project. For Authorized redirect URIs simply add /signin-google at the end of your JavaScript origin.
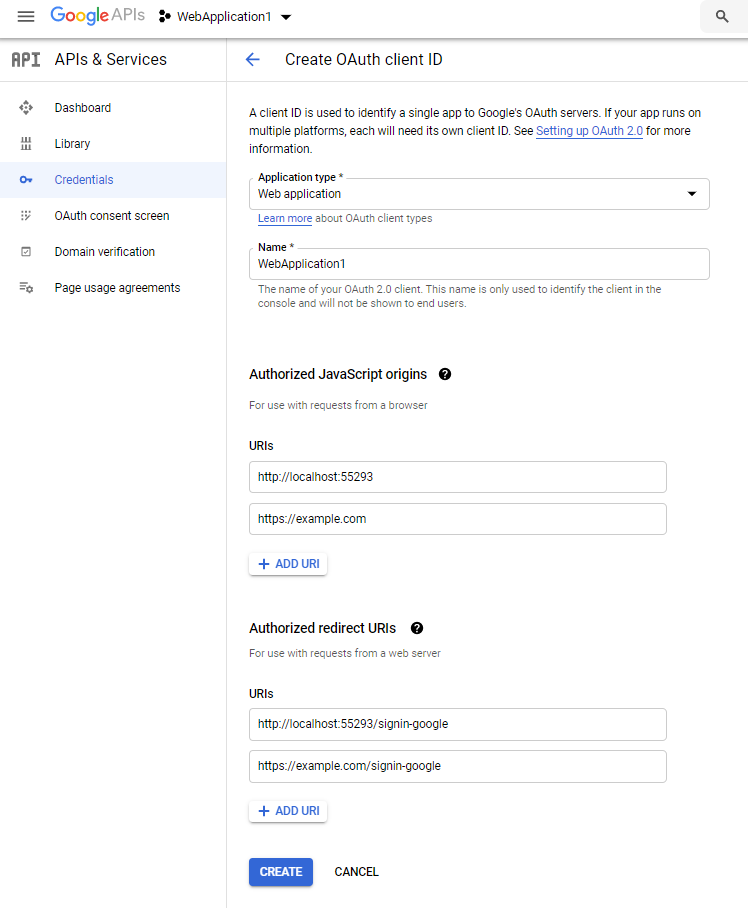
Click on create. Note down your Client ID and your Client Secret and save it securely.
STEP 4 Initialize Owin Security
Owin is a library by Microsoft that handles common types of several authentication middleware components like G-Suite authentication. To initialize this library, right-click on your project in Visual Studio, Add: New Item, and add OWIN Startup class.
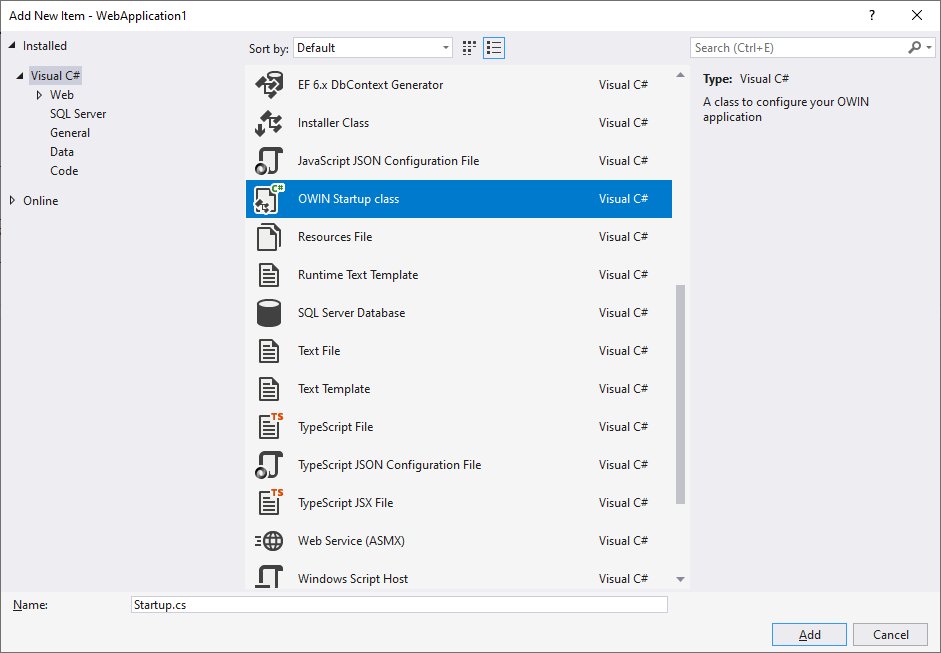
Within the Configuration method in the Startup class, provide your Client ID and your Client Secret generated in Step 2. The overall code looks like this:
using Microsoft.Owin; using Microsoft.Owin.Security.Google; using Owin; [assembly: OwinStartup(typeof(WebApplication1.Startup))] namespace WebApplication1 { public class Startup { public void Configuration(IAppBuilder app) { app.UseExternalSignInCookie("ExternalCookie"); GoogleOAuth2AuthenticationOptions options = new GoogleOAuth2AuthenticationOptions { ClientId = "1253456789012-exampleid9876543210exampleid.apps.googleusercontent.com", ClientSecret = "AbCdEfGhIjKlMnOpQrStUvWxYz", }; app.UseGoogleAuthentication(options); } } }
Do not change the namespace, class name or method name in this file. To know more about Owin Initialization, read its documentation here.
STEP 5 In your ASP.NET form, add G-Suite Authentication code
This is the most important part. This is where you will authenticate your users. To keep it simple, I’ve used a single page application with just one webform – Default.aspx.
In Default.aspx.cs Page_Load
method, you need to write the logic for authentication. Request.GetOwinContext().Authentication.GetExternalLoginInfo()
gives you the current ExternalLoginInfo
of the currently authenticated user. If this object is null
, redirect the user to the G-Suite authentication page by calling the Request.GetOwinContext().Authentication.Challenge
API. When this object is non-null, then you can get the information about the authenticated user from this object. If any error occurs during the authentication, the error message can be obtained using Request.QueryString["error"]
. The overall code for Default.aspx.cs looks like this:
using Microsoft.AspNet.Identity.Owin; using Microsoft.Owin.Security; using System; using System.Web; namespace WebApplication1 { public partial class Default : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { string ErrorMessage = Request.QueryString["error"]; if(string.IsNullOrWhiteSpace(ErrorMessage)) { ExternalLoginInfo LoginInfo = Request.GetOwinContext().Authentication.GetExternalLoginInfo(); if(LoginInfo==null) { Request.GetOwinContext().Authentication.Challenge( new AuthenticationProperties { RedirectUri = "/" }, new string[] { "Google" }); } else { WebMsg.InnerText = "G-Suite Login is Successful. Email=" + LoginInfo.Email; } } else { WebMsg.InnerText = "Cannot Login: " + ErrorMessage; } } } }
In addition, you should have <div runat="server" id="WebMsg"></div>
in your Default.aspx HTML to display success and error messages.
NOTE: If you are debugging in your local environment using Chrome, you might get an error “access_denied” on successful authentication. For instance, I have observed this while debugging over HTTP in newer versions of Chrome. Alternatively, you can use Firefox for debugging. The authentication works perfectly fine on Chrome when deployed to production.
Short and nice. Keep helping others pass it on.
How do I validate the user on a different web form? I have multiple aspx pages.
You can use the same API on your other web forms as well. Request.GetOwinContext().Authentication.GetExternalLoginInfo() will provide you ExternalLoginInfo till the session is valid. If ExternalLoginInfo is null, you can redirect your user to the login page.