Selenium is a browser automation tool to test web applications. It is used to automate the regular tasks of testing which allows the tester to focus on new feature tests.
Selenium supports various browsers like Firefox, Chrome, Safari, Opera, and Internet Explorer. To use the Selenium framework for automated testing, two things are required – the Selenium FrameWork and a suitable driver for your chosen browser.
Selenium requires a platform-dependent driver file to be placed between Selenium scripts and the browser to perform automation tasks.
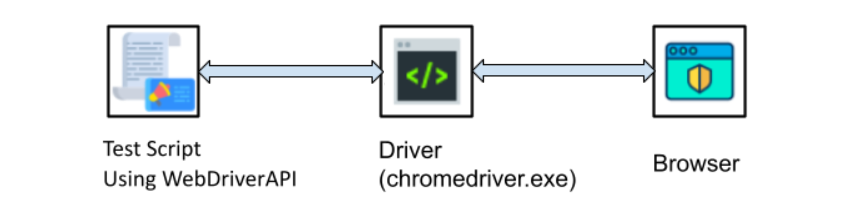
Challenges of Manual Driver Management
Manually downloading a driver executable for specific browsers like chromedriver.exe (for chrome), geckodriver.exe (for firefox), etc. with respect to operating systems (like Windows, Linux, or Mac OS) can be complicated. Moreover, the driver version should be compatible with the installed browser version. Keeping driver files compatible with the browser is quite a tedious task. If versions are incompatible then the test will fail.
Manual Driver Setup in a test script
We need to provide a driver file path in our Selenium scripts. Using Java system class we can set the driver path in selenium script.
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver");
For example: System.setProperty("webdriver.gecko.driver", "E:\Automation_Setup_Files\chromedriver.exe");
To overcome these disadvantages, we can implement WebDriverManager for managing our driver executables.
What is WebDriverManager?
WebDriverManager is a tool that automatically handles the download, setup, and management of Selenium WebDriver drivers (e.g., chromedriver.exe, geckodriver.exe, msedgedriver.exe, etc.)
WebDriverManager Setup
WebdriverManager is primarily used as a Java dependency. In Maven, this can be done by adding below dependency in pom.xml file:
<dependency>
<groupId>io.github.bonigarcia</groupId>
<artifactId>webdrivermanager</artifactId>
<version>5.0.3</version>
</dependency>
The following example demonstrates the use of WebDriverManager and Selenium Java.
package selenium.webDriverManager;
import java.time.Duration;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import io.github.bonigarcia.wdm.WebDriverManager;
public class WebDriverManagerChromeDriver {
public static void main(String[] args) {
WebDriver driver;
WebDriverManager.chromedriver().setup();
driver = new ChromeDriver();
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(Duration.ofMillis(60));
driver.get("https://google.com");
String title = driver.getTitle();
System.out.println("Webiste title is: " + title);
driver.quit();
}
}
Note:
WebDrivermanager provides managers for all major browsers. We can invoke WebDriverManagers by using the below commands:
WebDriverManager.chromedriver().setup();
WebDriverManager.firefoxdriver().setup();
WebDriverManager.edgedriver().setup();
WebDriverManager.chromiumdriver().setup()
We can implement WebDriverManager with any design pattern and automation framework.