In this blog, we will see how to delete an object in S3 using ASP .NET Core. For this, we need to have some objects in S3 bucket. Adding objects to S3 is explained in the previous blog “Various Operations on S3 Bucket using ASP .NET Core Part-1” you can refer to the blog to Create folders/files in S3 Bucket.
Let’s try to delete a file in the “Files” Folder which has a .txt file named “File1.txt”.
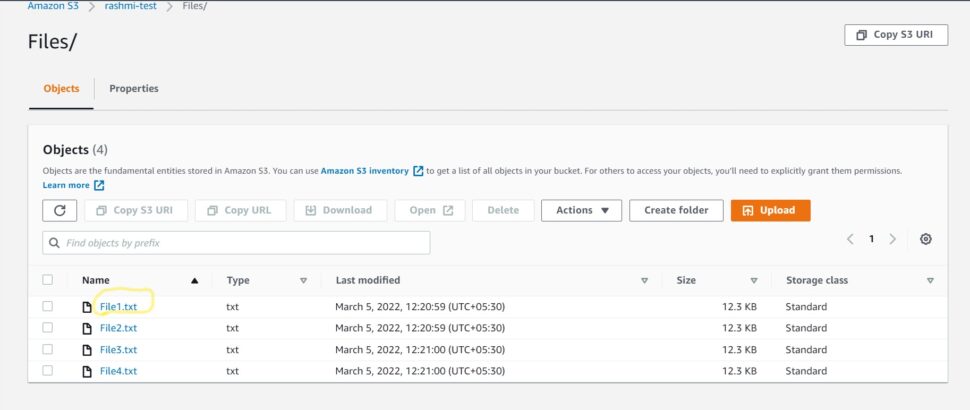
private static async Task<DeleteObjectsResponse> deleteObjects(List<DeleteObject> objectsToBeDeleted)
{
try
{
AmazonS3Client Client = new AmazonS3Client(PublicKey, SecretKey, Region);
var deleteObjectsRequest = new DeleteObjectsRequest();
deleteObjectsRequest.BucketName = BucketName;
foreach (DeleteObject obj in objectsToBeDeleted)
{
deleteObjectsRequest.AddKey(obj.Key);
}
var response = await Client.DeleteObjectsAsync(deleteObjectsRequest);
return response;
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
return null;
}
We are initializing the AmazonS3Client and passing the PublicKey, SecretKey, and Region, the values for these are accessed from appsetting.json.
Task<DeleteObjectsResponse> Using a single HTTP request, you can delete numerous objects from a bucket with this action. If you know the object keys that you want to delete, then this action provides a suitable alternative to sending individual delete requests, reducing pre-request overhead. A list of up to 1000 keys to delete is included in the request.
string deleteObjectsRequest.BucketName {get; set;} Gets and sets Property BucketName.
deleteObjectsRequest.AddKey(string key): Adds a key to the set of keys of object to be deleted.
In the catch block, we are catching the exception that occurs during application execution.
public static async Task<List<DeleteObject>> GetObjectsToBeDeleted()
{
AmazonS3Client Client = new AmazonS3Client(PublicKey, SecretKey, Region);
ListObjectsRequest Request = new ListObjectsRequest
{
BucketName = BucketName,
};
ListObjectsResponse Result;
List<DeleteObject> DeleteObjects = new List<DeleteObject>();
do
{
Result = await Client.ListObjectsAsync(Request);
foreach (S3Object o in Result.S3Objects)
{
if (o.Key.Contains(keyword))
{
DeleteObjects.Add(new DeleteObject
{
Size = (o.Size / 1024).ToString() + "KB",
Uploaded = o.LastModified,
Key = o.Key,
});
}
}
Console.WriteLine("Deleted" + keyword);
}
while (Result.IsTruncated);
Client.Dispose();
return DeleteObjects;
}
We are initializing the AmazonS3Client and passing the PublicKey, SecretKey, and Region, the values for these are accessed from appsetting.json
ListObjectsRequest Request: It is a container for the parameter to the ListObjects operations. This returns some or all of the objects in a bucket (up to 1000). To return a subset of the objects in a bucket, use the request parameters as selection criteria. Valid or invalid XML can be found in a 200 Ok response.
Then, we are using a loop in which we have S3Object, which contains all the attributes that S3 has. And if it contains the keyword that we are looking for it executes the if condition where it will check for Size, Last Modified date, and the keyword. Then we are printing the deleted keyword.
Result.IsTruncated {get; set;}: It is a flag that indicates whether or not Amazon S3 returned all of the results that satisfied the search criteria.
Client.Dispose(): This function performs application-defined tasks associated with freeing, releasing, or resetting unmanaged resources.
As you can see the file named File1 has been deleted.
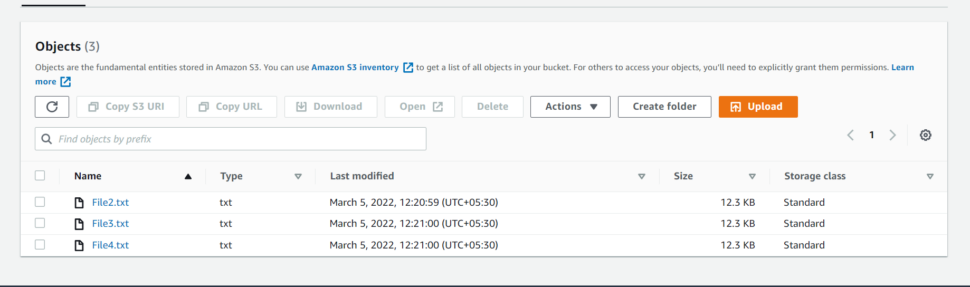
This is how we Delete an object in S3 Bucket using ASP .NET Core.