What is Cryptography?
Cryptography is that the art of communication between a sender and a receiver using codes in order that only those for whom the knowledge is meant can read and process it.
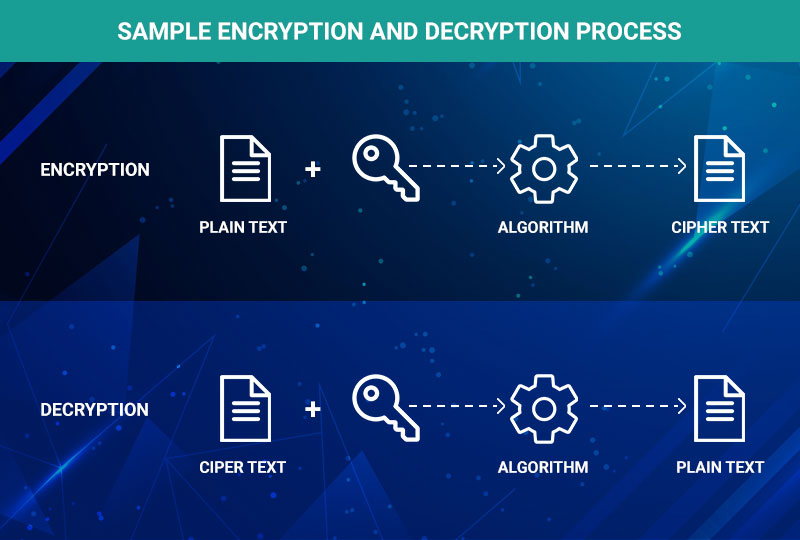
Terminologies of Cryptography
Plain Text: it is the text which is readable and can be understood by all users.
Cipher Text: the message obtained after applying cryptography on plain text is Cipher text.
Encryption: the method of converting plain text to cipher text is named encryption. It is also called encoding.
Decryption: the method of converting cipher text to plain text is named decryption. It is also termed decoding.
Why Encryption is important?
- For maintaining the security of financial and other Research & Development data with various internal data which needs to be safe from intruders.
- The employees or organizations don’t want their financial or private items to be made public.
- To make quick transformation so that private items are not made public.
Encryption scrambles text to make it unreadable by others and the security strategy should have the ability to slow down hackers from stealing sensitive information.
Installation Process
Step1: We need to install two libraries i.e., pip install pycrypto for decryption and pip install base32hex for base32 decoding (Note: pycrypto installation works for python 2.7.9 versions.) The PyCrypto package is the most well-known third-party cryptography package for Python. Sadly PyCrypto’s development stopping in 2012. Others have continued to release the newest version of PyCryto so you’ll still catch on for Python 3.5. Alternatively, there’s a fork of the project called PyCrytodome that’s a replacement for PyCrypto.
Do not Install PyCrypto and PyCryptodome at an equivalent time, as they’re going to interfere with one another.
From the command prompt, we need to install the required libraries using the following commands, Mac users can use pip3 instead of pip
pip install pycrypto
pip install base32hex
Step2: Import the following libraries.
- We are using base32hex to encode and decode the string.
- Hashlib module is an interface for hashing message. The idea behind this module is to use a hash function on a string, and encrypt in order that it’s very difficult to decrypt it.
The code for Encryption is mentioned below:
import base32hex
import hashlib
from Crypto.Cipher import DES
password = "Password"
salt = '\x28\xAB\xBC\xCD\xDE\xEF\x00\x33'
key = password + salt
m = hashlib.md5(key)
key = m.digest()
(dk, iv) =(key[:8], key[8:])
crypter = DES.new(dk, DES.MODE_CBC, iv)
plain_text= "I see you"
print("The plain text is : ",plain_text)
plain_text += '\x00' * (8 - len(plain_text) % 8)
ciphertext = crypter.encrypt(plain_text)
encode_string= base32hex.b32encode(ciphertext)
print("The encoded string is : ",encode_string)
Output after Encryption

The code for Decryption is mentioned below:
import base32hex
import hashlib
from Crypto.Cipher import DES
password = "Password"
salt = '\x28\xAB\xBC\xCD\xDE\xEF\x00\x33'
key = password + salt
m = hashlib.md5(key)
key = m.digest()
(dk, iv) =(key[:8], key[8:])
crypter = DES.new(dk, DES.MODE_CBC, iv)
encrypted_string='UH562EGM8RCHHTOUC5CTRS59OG======'
print("The ecrypted string is : ",encrypted_string)
encrypted_string=base32hex.b32decode(encrypted_string)
decrypted_string = crypter.decrypt(encrypted_string)
print("The decrypted string is : ",decrypted_string)
Output after Decryption
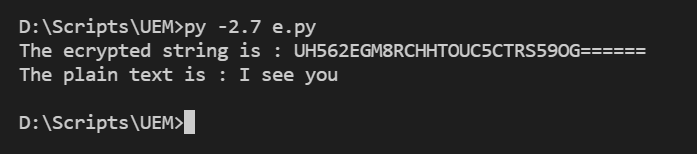
This is the procedure to decrypt using pycrypto Python Library using salt, password and key.
cool
–
March 4, 2022Thank you!
How did you obtain encrypted string in the decryption code?
–
March 4, 2022I have initialized encrypted string in the Decryption code to see the before and after results.
Very helpful 👍👍
explained well
Great initiative
Why Encryption is important?
For maintaining the security of financial and other Research & Development data with various internal data which needs to be safe from intruders.
The employees or organizations don’t want their financial or private items to be made public.
To make quick transformation so that private items are not made public.
Encryption scrambles text to make it unreadable by others and the security strategy should have the ability to slow down hackers from stealing sensitive information.
Installation Process
Step1: We need to install two libraries i.e., pip install pycrypto for decryption and pip install base32hex for base32 decoding (Note: pycrypto installation works for python 2.7.9 versions.) The PyCrypto package is the most well-known third-party cryptography package for Python. Sadly PyCrypto’s development stopping in 2012. Others have continued to release the newest version of PyCryto so you’ll still catch on for Python 3.5. Alternatively, there’s a fork of the project called PyCrytodome that’s a replacement for PyCrypto.
Do not Install PyCrypto and PyCryptodome at an equivalent time, as they’re going to interfere with one another.
From the command prompt, we need to install the required libraries using the following commands,