What is MVVM?
MVVM stands for Model View ViewModel. It’s used in the software industry because it helps to write different types of logic as a business, UI logic in different modules, and popular approaches in the modern software industry.
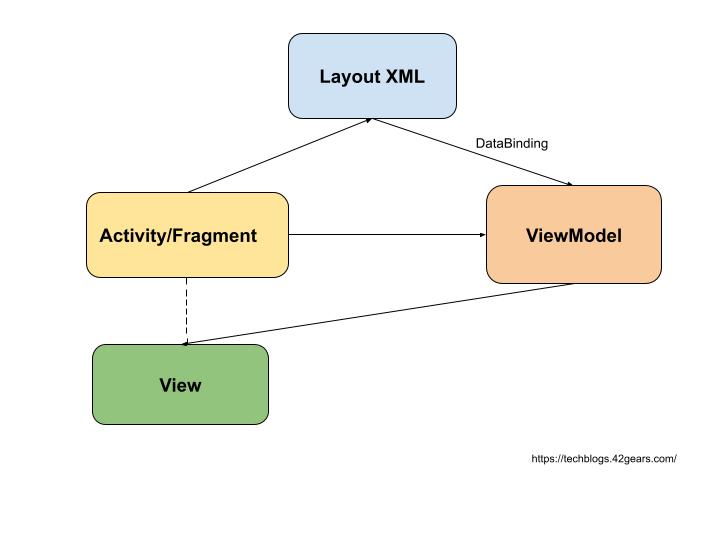
Model: Basically model means the object which holds data that we need to display in UI or used for the business layout to make some calculations.
package com.gears42.databindingkotlin data class DataBindingKotlinModel(val fName: String, val lName: String)
View: View basically is UI elements that will display data to end-user such as TextView, CheckBox, Button etc.
<?xml version="1.0" encoding="utf-8"?> <layout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools"> <data> <variable name="model" type="com.gears42.databindingkotlin.DataBindingKotlinModel" /> </data> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <TextView android:id="@+id/user_name_text_view" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="16dp" android:text="@{model.fName}" tools:text="Name" /> <TextView android:id="@+id/user_age_text_view" android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="16dp" android:text="@{model.lName}" tools:text="XX" /> </LinearLayout> </layout>
ViewModel
it’s a link between Model & View, where we can add Business logic and some of the display logic in it, it’s a mater piece of MVVM.
What is Data Binding?
It’s a Library Offer by Google while I/O 2015. It makes developer life easy to main clean code. we will use ActivityBiding in this blogs, in case of Fragment we can use DataBindingUtil.inflate
package com.gears42.databindingkotlin import androidx.databinding.DataBindingUtil import android.os.Bundle import androidx.appcompat.app.AppCompatActivity import com.gears42.databindingkotlin.databinding.ActivityMainBinding class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) var binding: ActivityMainBinding = DataBindingUtil.setContentView(this, R.layout.activity_main) var user = DataBindingKotlinModel("Imtiyaz", "Khalani") binding.setVariable(BR.model, user) binding.executePendingBindings() } }
output of the above code.
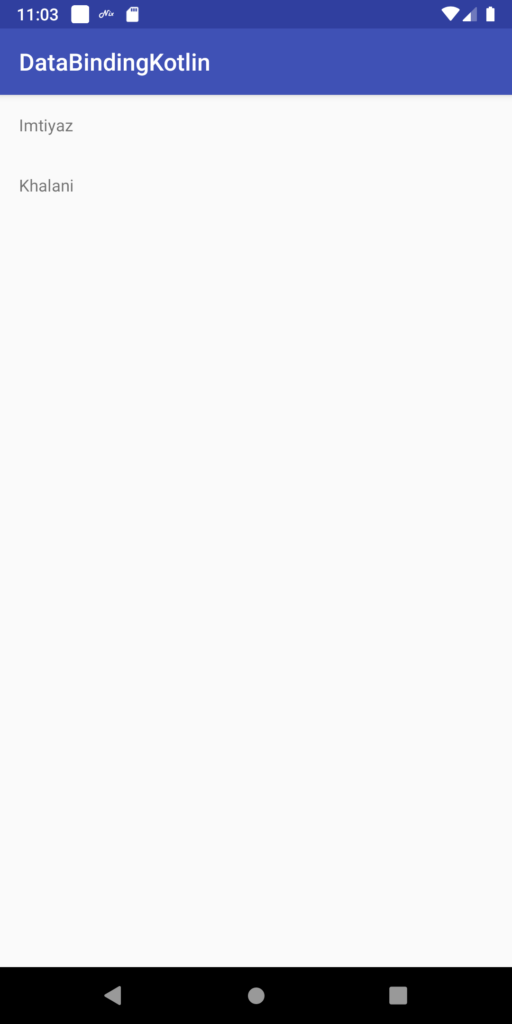
Download or check out the entire source code please check Github. this repo includes another example as well how to use along with Fragment, with two-way data binding.
Read more blogs on 42Gears TechBlog.