Let’s see how to list objects i.e files and folders of S3 Bucket using ASP .NET Core, we have seen how to List the Buckets in Amazon S3 using ASP .NET Core in Part-2
Note: Files and folders in S3 buckets are known as Objects.
We will try to list all the files and folders known as objects present in AWS S3 using ASP .NET Core. For example, I have three folders namely “Files” which contains .txt files, “Sheets” which contain .csv files, and “Reports” which contain both .txt and .csv files.
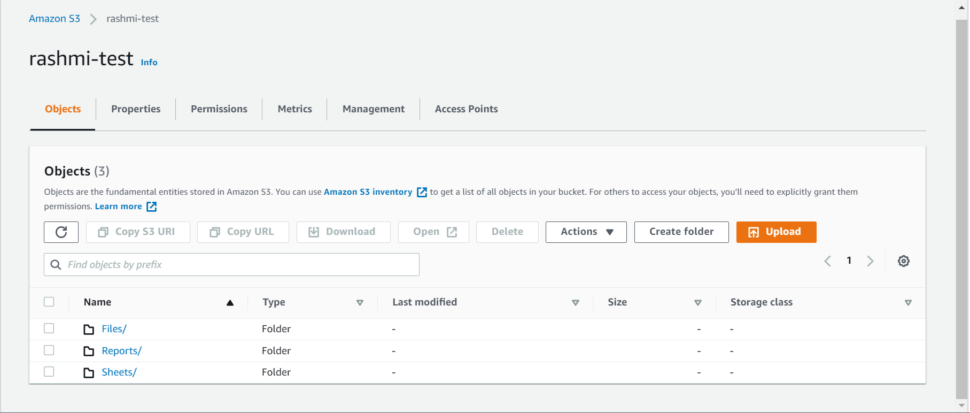
Let’s just try to open one of the folders and see the content in that folder
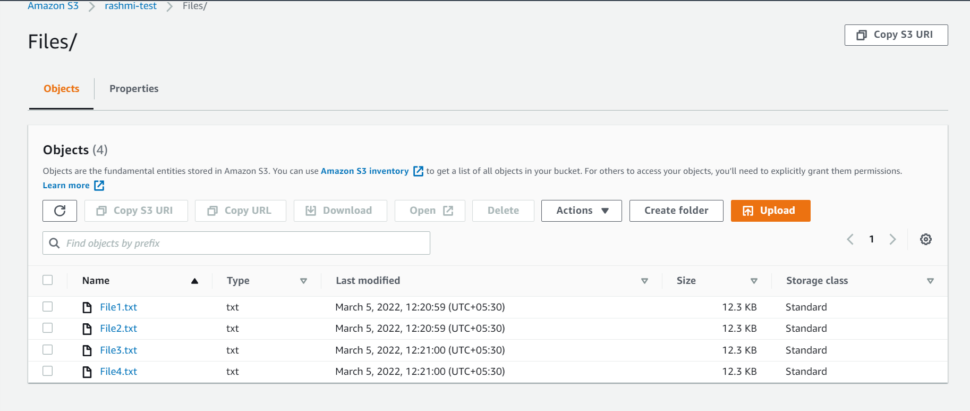
public static async Task ListObjects()
{
AmazonS3Client Client = new AmazonS3Client(PublicKey, SecretKey, Region);
ListObjectsRequest listObjectsRequest = new ListObjectsRequest();
listObjectsRequest.BucketName = BucketName;
listObjectsRequest.MaxKeys = 2;
int i = 1;
ListObjectsResponse listObjectsResponse = await Client.ListObjectsAsync(listObjectsRequest);
do
{
List<S3Object> s3Objects = listObjectsResponse.S3Objects;
foreach (S3Object s3Object in s3Objects)
{
Console.WriteLine("Key " + s3Object.Key);
}
Console.WriteLine("Request " + (++i));
listObjectsRequest.Marker = listObjectsResponse.NextMarker;
listObjectsResponse = await Client.ListObjectsAsync(listObjectsRequest);
}
while (listObjectsResponse.IsTruncated);
}
We are initializing the AmazonS3Client and passing the PublicKey, SecretKey, and Region, the values for these are accessed from appsetting.json. In the next step, we are creating a list of Bucket using the function ListBucketsAsync(). string ListObjectsRequest.BucketName {get; set;} gets and sets the property BucketName. The name of Bucket containing the objects.
The class ListObjectsRequest is a container for parameters to the ListObject operation. It returns some or all (up to 1000) objects in a bucket. You can use the request parameters as the selection criteria to return a subset of the objects in a bucket. A 200 Okay response can contain valid or invalid XML.
The int listObjectsRequest.MaxKeys {get; set;} gets and sets the property MaxKeys. This sets the utmost number of keys returned within the response. But, by default, the action returns up to 1000 key names. The response could contain fewer keys but it will never contain more.
string listObjectsRequest.Marker {get; set;} gets and sets the property Marker. Marker is where you want Amazon S3 to begin listing from. Following this specific key, Amazon S3 begins listing. Marker is often any key within the bucket.
string listObjectsResponse.NextMarker {get; set;} gets and sets the NextMarker property. Next Marker is set by S3 only if a Delimiter was specified in the original listObjects request. If a delimiter was not specified, the AWS SDK for .NET returns the last Key of the List of Object retrieved from S3 as the NextMarker.
bool listObjectsResponse.IsTruncated {get; set;} is a flag that indicates whether or not Amazon S3 returned all of the results that satisfied the search criteria.
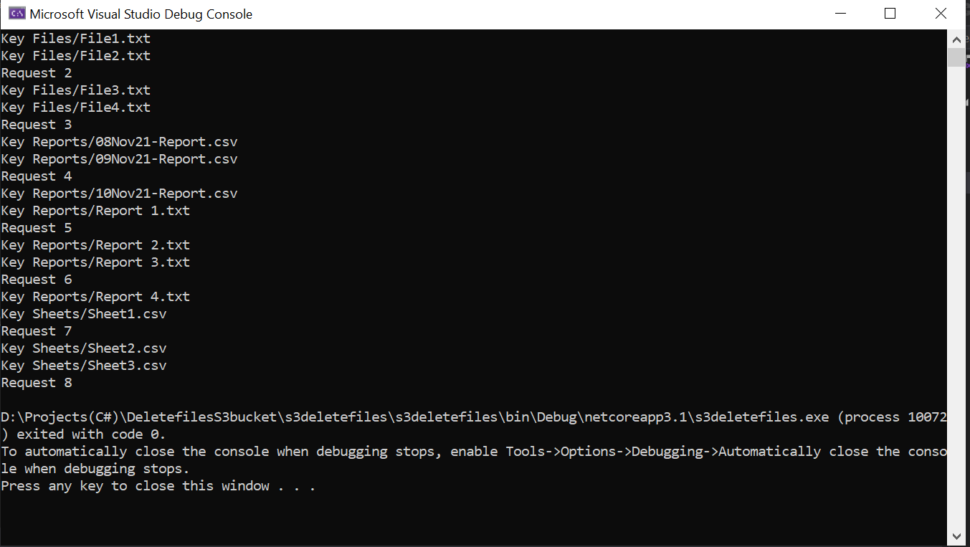
This is how we List objects in Amazon S3 Bucket using ASP .NET Core. In the next blog, we will see how to Delete an object using ASP .NET Core.